DirtyLude
Well-Known Member
I just wanted to post a quick note on this sensor because it's pretty cool. I bought one to add to a datalogging system I'm going to use while canoeing. It'll have GPS, Pressure, Temperature, and Humidity. That's all that I could think of. So I bought this sensor and made up a little board for it.
The sensor has a thermometer and pressure sensor in it and the temperature is used to compensate the pressure data. It doesn't do this itself, you have to do it. There is also a bunch of encoded calibration data in the chip itself you need to take into account. So there's a bunch of coding you'll need to do on your microcontroller.
I just pulled all the calculations from these arduino sites where the code is simply based off the datasheet, but slightly cleaned up. Looks like you can get a breakout board from the first link; second link shows that if you are pretty careful you can directly solder some small gauge wires. You only need a few connections.
**broken link removed**
**broken link removed**
Anyway, the thing can really tell the difference in pressure every few feet, even at its lowest resolution. Here it is showing the data its getting. The temperature is in degrees Celsius. I didn't bother putting in a decimal point, it's 23.2 degrees. Fairly warm. Temperature data seems much more sensitive than the LM75. Putting my finger on it, it immediately jumps several degrees, very quickly. The Pressure is shown in Pascals and it's about 20 Pascals difference in the Pressure at my feet compared to at my head height.
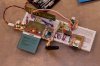
Here is the code I used for calculations, but it's pretty much the same as in the linked pages.
First you have to get all the calibration data stored in ROM. You only need to do this once.
Then you retrieve the uncompensated raw temp and pressure data from the sensor and use them in these calculations.
This is a better picture of the sensor mounted.
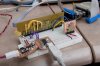
The sensor has a thermometer and pressure sensor in it and the temperature is used to compensate the pressure data. It doesn't do this itself, you have to do it. There is also a bunch of encoded calibration data in the chip itself you need to take into account. So there's a bunch of coding you'll need to do on your microcontroller.
I just pulled all the calculations from these arduino sites where the code is simply based off the datasheet, but slightly cleaned up. Looks like you can get a breakout board from the first link; second link shows that if you are pretty careful you can directly solder some small gauge wires. You only need a few connections.
**broken link removed**
**broken link removed**
Anyway, the thing can really tell the difference in pressure every few feet, even at its lowest resolution. Here it is showing the data its getting. The temperature is in degrees Celsius. I didn't bother putting in a decimal point, it's 23.2 degrees. Fairly warm. Temperature data seems much more sensitive than the LM75. Putting my finger on it, it immediately jumps several degrees, very quickly. The Pressure is shown in Pascals and it's about 20 Pascals difference in the Pressure at my feet compared to at my head height.
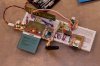
Here is the code I used for calculations, but it's pretty much the same as in the linked pages.
First you have to get all the calibration data stored in ROM. You only need to do this once.
Code:
I2CWriteLength = 2;
I2CReadLength = 22;
I2CMasterBuffer[0] = BMP085_ADDR;
I2CMasterBuffer[1] = 0xAA;
I2CMasterBuffer[2] = BMP085_ADDR | RD_BIT;
I2CEngine();
ac1 = (I2CSlaveBuffer[0] << 8) | I2CSlaveBuffer[1];
ac2 = (I2CSlaveBuffer[2] << 8) | I2CSlaveBuffer[3];
ac3 = (I2CSlaveBuffer[4] << 8) | I2CSlaveBuffer[5];
ac4 = (I2CSlaveBuffer[6] << 8) | I2CSlaveBuffer[7];
ac5 = (I2CSlaveBuffer[8] << 8) | I2CSlaveBuffer[9];
ac6 = (I2CSlaveBuffer[10] << 8) | I2CSlaveBuffer[11];
b1 = (I2CSlaveBuffer[12] << 8) | I2CSlaveBuffer[13];
b2 = (I2CSlaveBuffer[14] << 8) | I2CSlaveBuffer[15];
mb = (I2CSlaveBuffer[16] << 8) | I2CSlaveBuffer[17];
mc = (I2CSlaveBuffer[18] << 8) | I2CSlaveBuffer[19];
md = (I2CSlaveBuffer[20] << 8) | I2CSlaveBuffer[22];
Then you retrieve the uncompensated raw temp and pressure data from the sensor and use them in these calculations.
Code:
//Retrieve the Uncompensated Termperature (ut) and the Uncompensated Pressure (up)
//This code has been cut out. Just standard I2C data retrieve
//calculate the temperature
x1 = (ut - ac6) * ac5 >> 15;
x2 = ((int32_t) mc << 11) / (x1 + md);
b5 = x1 + x2;
temperature = (b5 + 8) >> 4;
//calculate the pressure
b6 = b5 - 4000;
x1 = (b2 * (b6 * b6 >> 12)) >> 11;
x2 = ac2 * b6 >> 11;
x3 = x1 + x2;
b3 = ((int32_t) ac1 * 4 + x3 + 2) >> 2;
x1 = ac3 * b6 >> 13;
x2 = (b1 * (b6 * b6 >> 12)) >> 16;
x3 = ((x1 + x2) + 2) >> 2;
b4 = (ac4 * (uint32_t) (x3 + 32768)) >> 15;
b7 = ((uint32_t) up - b3) * 50000;
p = b7 < 0x80000000 ? (b7 * 2) / b4 : (b7 / b4) * 2;
x1 = (p >> 8) * (p >> 8);
x1 = (x1 * 3038) >> 16;
x2 = (-7357 * p) >> 16;
pressure = p + ((x1 + x2 + 3791) >> 4);
This is a better picture of the sensor mounted.
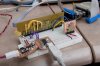
Last edited: