I am trying to send a string via 433 MHz transmitter (DigiKey 1597-1223-ND) attached to a Pro-Micro 5V (https://www.sparkfun.com/products/12640) to a receiver attached to Raspberry Pi 3.
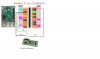
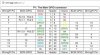
I am using the RadioHead Library to send the transmission from the Pro-Micro to the transmitter found from this tutorial https://randomnerdtutorials.com/rf-433mhz-transmitter-receiver-module-with-arduino/.
I am using WiringPi and 433Utils to receive the transmission from the receiver to Raspberry Pi 3 using this tutorial https://www.princetronics.com/how-to-read-433-mhz-codes-w-raspberry-pi-433-mhz-receiver/.
I think the sniffer code is working but the Raspberry Pi does not appear to be receiving anything even when I place the receiver and transmitter right next to each other. The Putty console looks like this once the code is running.
Please help me understand why the Putty console of the Raspberry Pi is not displaying the transmission.
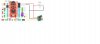
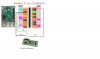
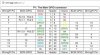
I am using the RadioHead Library to send the transmission from the Pro-Micro to the transmitter found from this tutorial https://randomnerdtutorials.com/rf-433mhz-transmitter-receiver-module-with-arduino/.
Code:
#include <RH_ASK.h>
#include <SPI.h> // Not actually used but needed to compile
RH_ASK driver;
void setup()
{
Serial.begin(9600); // Debugging only
if (!driver.init())
Serial.println("init failed");
}
void loop()
{
const char *msg = "Hello World!";
driver.send((uint8_t *)msg, strlen(msg));
driver.waitPacketSent();
delay(1000);
}
I am using WiringPi and 433Utils to receive the transmission from the receiver to Raspberry Pi 3 using this tutorial https://www.princetronics.com/how-to-read-433-mhz-codes-w-raspberry-pi-433-mhz-receiver/.
Code:
/*
RFSniffer
Usage: ./RFSniffer [<pulseLength>]
[] = optional
Hacked from http://code.google.com/p/rc-switch/
by @justy to provide a handy RF code sniffer
*/
#include "../rc-switch/RCSwitch.h"
#include <stdlib.h>
#include <stdio.h>
RCSwitch mySwitch;
int main(int argc, char *argv[]) {
// This pin is not the first pin on the RPi GPIO header!
// Consult https://projects.drogon.net/raspberry-pi/wiringpi/pins/
// for more information.
int PIN = 2;
if(wiringPiSetup() == -1) {
printf("wiringPiSetup failed, exiting...");
return 0;
}
int pulseLength = 0;
if (argv[1] != NULL) pulseLength = atoi(argv[1]);
mySwitch = RCSwitch();
if (pulseLength != 0) mySwitch.setPulseLength(pulseLength);
mySwitch.enableReceive(PIN); // Receiver on interrupt 0 => that is pin #2
while(1) {
if (mySwitch.available()) {
int value = mySwitch.getReceivedValue();
if (value == 0) {
printf("Unknown encoding\n");
} else {
printf("Received %i\n", mySwitch.getReceivedValue() );
}
mySwitch.resetAvailable();
}
}
exit(0);
}
I think the sniffer code is working but the Raspberry Pi does not appear to be receiving anything even when I place the receiver and transmitter right next to each other. The Putty console looks like this once the code is running.
Please help me understand why the Putty console of the Raspberry Pi is not displaying the transmission.