GettinBetter
New Member
H/W: EastPIC V7 Dev Board, ICD3, WDG0151-TMI GLCD, PIC16DF877, Salea16 Analyser.
S/W: MPLABX, XC8,
I have the following example code slightly modified to suit my application, but it's not quite there yet, hopefully it's something simple that you guys might spot.
The EasyPIC board has a pot directly connected that allows contrast to be adjusted, works fine in that I can brighten and darken the pixels, but alas not characters will appear.
Ant pointers would be appreciated
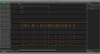
S/W: MPLABX, XC8,
I have the following example code slightly modified to suit my application, but it's not quite there yet, hopefully it's something simple that you guys might spot.
The EasyPIC board has a pot directly connected that allows contrast to be adjusted, works fine in that I can brighten and darken the pixels, but alas not characters will appear.
Ant pointers would be appreciated
C:
/*
* File: main.c
* Author: Example Code from exploreembedded.com
* Created on 09 January 2021, 23:37
* Project name: Testing GLCD with PIC16F877
*/
// CONFIG
#pragma config FOSC = XT // Oscillator Selection bits (XT oscillator)
#pragma config WDTE = OFF // Watchdog Timer Enable bit (WDT disabled)
#pragma config PWRTE = OFF // Power-up Timer Enable bit (PWRT disabled)
#pragma config CP = OFF // FLASH Program Memory Code Protection bits (Code protection off)
#pragma config BOREN = ON // Brown-out Reset Enable bit (BOR enabled)
#pragma config LVP = OFF // Low Voltage In-Circuit Serial Programming Enable bit (RB3 is digital I/O, HV on MCLR must be used for programming)
#pragma config CPD = OFF // Data EE Memory Code Protection (Code Protection off)
#pragma config WRT = ON // FLASH Program Memory Write Enable (Unprotected program memory may be written to by EECON control)
#include <xc.h>
#define _XTAL_FREQ 4000000L
/**
* Control bus PB0:PB4
* Data bus PD0:PD7
*/
#define GlcdDataBus PORTD
#define GlcdControlBus PORTB
#define GlcdDataBusDirnReg TRISD
#define GlcdCtrlBusDirnReg TRISB
#define CS1 0x00 // Select segments 1-64
#define CS2 0x01 // Select segments 65-128
#define RS 0x02 // H: Data - L: Instruction
#define RW 0x03 // H: Read Data - L: Write Data
#define EN 0x04 // Enable
/* 5x7 Font including 1 space to display HELLO WORLD */
char H[]={0x7F, 0x08, 0x08, 0x08, 0x7F, 0x00};
char E[]={0x7F, 0x49, 0x49, 0x49, 0x41, 0x00};
char L[]={0x7F, 0x40, 0x40, 0x40, 0x40, 0x00};
char O[]={0x3E, 0x41, 0x41, 0x41, 0x3E, 0x00};
char W[]={0x3F, 0x40, 0x38, 0x40, 0x3F, 0x00};
char R[]={0x7F, 0x09, 0x19, 0x29, 0x46, 0x00};
char D[]={0x7F, 0x41, 0x41, 0x22, 0x1C, 0x00};
void Glcd_SelectPage0() // CS1=1, CS2=0
{
GlcdControlBus |= (1<<CS1);
GlcdControlBus &= ~(1<<CS2);
}
void Glcd_SelectPage1() // CS1=0, CS1=1
{
GlcdControlBus &= ~(1<<CS1);
GlcdControlBus |= (1<<CS2);
}
/* Function to send the command to LCD */
void Glcd_CmdWrite(char cmd)
{
GlcdControlBus |= (1<<EN); // Generate a High-to-low pulse on EN pin
__delay_us(500);
GlcdDataBus = cmd; //Send the Command byte
GlcdControlBus &= ~(1<<EN); // Bring the EN pin low again
GlcdControlBus &= ~(1<<RS); // Send LOW pulse on RS pin for selecting Command register
GlcdControlBus &= ~(1<<RW); // Send LOW pulse on RW pin for Write operation
__delay_us(1000);
}
/* Function to send the Data to LCD */
void Glcd_DataWrite(char dat)
{
GlcdControlBus |= (1<<EN); // Generate a High-to-low pulse on EN pin
__delay_us(500);
GlcdDataBus = dat; //Send the data on DataBus byte
GlcdControlBus &= ~(1<<EN); // Bring the EN pin low again
GlcdControlBus |= (1<<RS); // Send HIGH pulse on RS pin for selecting data register
GlcdControlBus &= ~(1<<RW); // Send LOW pulse on RW pin for Write operation
__delay_us(1000);
}
void Glcd_DisplayChar(char *ptr_array)
{
int i;
for(i=0;i<6;i++) // 5x7 font, 5 chars + 1 blank space
Glcd_DataWrite(ptr_array[i]);
}
int main()
{
GlcdDataBusDirnReg = 0x00; // Configure all the Data (PORTD) pins as output
GlcdCtrlBusDirnReg = 0x00; // Configure the Ctrl (PORTB) pins as output
/* Select the Page0/Page1 and Turn on the GLCD */
Glcd_SelectPage0(); // CS1=1, CS2=0
Glcd_CmdWrite(0x3F); // Display ON
Glcd_SelectPage1(); // CS1=0, CS1=1
Glcd_CmdWrite(0x3F); // Display ON
__delay_us(500);
/* Select the Page0/Page1 and Enable the GLCD */
Glcd_SelectPage0(); // CS1=1, CS2=0
Glcd_CmdWrite(0xC0); //
Glcd_SelectPage1(); // CS1=0, CS1=1
Glcd_CmdWrite(0xC0); //
__delay_us(500);
Glcd_SelectPage0(); // Display HELLO on Page0, Line1
Glcd_CmdWrite(0xB8); // 0xB8 = line 0 (the top line)
Glcd_DisplayChar(H);
Glcd_DisplayChar(E);
Glcd_DisplayChar(L);
Glcd_DisplayChar(L);
Glcd_DisplayChar(O);
Glcd_SelectPage1(); // Display WORLD on Page1, Last line
Glcd_CmdWrite(0xBF); // 0xB8 = line 7 (the bottom line)
Glcd_DisplayChar(W);
Glcd_DisplayChar(O);
Glcd_DisplayChar(R);
Glcd_DisplayChar(L);
Glcd_DisplayChar(D);
while(1);
}
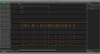
Last edited: